Pagination
For resources that return an undetermined amount of records, the ability to use paging has been implemented. Any resource with a {page} and {pageSize} parameter can implement paging.
- The {pageSize} determines the amount of records to pull for a given page.
- The {page} parameter determines which particular subset records to retrieve given the {pageSize}.
- If {page} or {pageSize} is 0, all records will be returned.
If the following route is called: api/violationcategories?page=2&pageSize=5
The 6th, 7th, 8th, 9th, and 10th category records will be returned.
Other examples:
- api/violationcategories?page=0&pageSize=0 - returns all records, no paging
- api/violationcategories - returns all records, no paging
- api/violationcategories?page=1&pageSize=1 - returns only the very first record
- api/violationcategories?page=5&pageSize=50 - returns the 200th through 250th record
Sorting
Any resource with a sort parameter can implement sorting. Each route allows specific sorting options wich are explained in details in the route's help.
- The sort parameter take a list of comma separated fields, each with a possible unary negative to imply descending sort order.
- Unary positive is the default sort direction used, which implies ascending order.
- Each route that allows sorting defaults to some order which is specified in the route's help.
Violations will be returned sorted in ascending order of DateCreated
Other examples:
- api/violations?sort=+datecreated - returns same results as api/violations?sort=datecreated
- api/violations?sort=-datecreated - returns violations sorted in descending order of DateCreated
- api/violations?sort=unitdisplayorder,-datecreated - returns violations sorted in ascending order of UnitDisplayOrder.
Violations with same UnitDisplayOrder will be sorted in descending order of DateCreated
File Download
To retrieve a physical file (such as an image or PDF), there is a two-step process.
- Call the GET method of the desired resource. Each resource that has a physical file will contain a property in their model called PathToFile. When receiving the JSON data, the PathToFile fied will have an encoded path to the desired file.
- Call the GetFile resource and pass in the PathToFile value in a query string parameter called filePath. This method will decode the path and retrieve the file from our file system. It will then load it into the response object with the proper content type.
- Call the violation photo GET method (api/violationphotos/1234). The violation photo JSON will be returned with the field PathToFile, which will have a value that looks something like: continental01%5c30%5cWorkOrders%5c2014822162712750_638208377.jpg
- Call the GetFile resource with the PathToFile value (api/files?filePath=continental01%5c30%5cWorkOrders%5c2014822162712750_638208377.jpg)
Documents
- api/documents/{Id}
- api/properties/{PropertyId}/documents?documentTypeId={documentTypeId}&documentSubTypeId={documentSubTypeId}&startDate={startDate}&endDate={endDate}&page={page}&pageSize={pageSize}
- api/violationphotos/{Id}
- api/violations/{ViolationId}/violationphotos?page={page}&pageSize={pageSize}
- api/properties/{PropertyId}/violationphotos?page={page}&pageSize={pageSize}
- api/units/{UnitId}/violationphotos?page={page}&pageSize={pageSize}
- api/violations/{ViolationId}/violationletters?page={page}&pageSize={pageSize}
- api/properties/{PropertyId}/violationletters?page={page}&pageSize={pageSize}
- api/workorderphotos/{Id}
- api/workorders/{WorkOrderId}/workorderphotos?page={page}&pageSize={pageSize}
- api/properties/{PropertyId}/workorderphotos?page={page}&pageSize={pageSize}
File Upload
To upload a physical file, the following steps must be followed:
- The POST resource of the desired module must be called.
For example, to insert a violation photo, the api/violationphotos route must be called using http POST.
- The content type must be set as multipart/form-data. In the request header, "Content-Type: multipart/form-data" must be present.
- The request body must contain all form data.
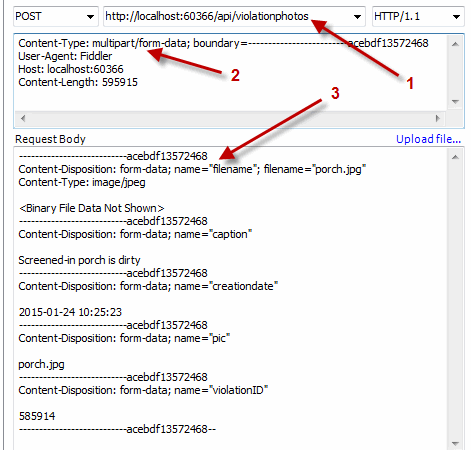
The following is a complete list of routes where a file can be uploaded and the directions above must be applied:
- Violation Photos: api/violationphotos
- Work Order Photos: api/workorderphotos